- Llambduh's Newsletter
- Posts
- EC2 Free Tier Management with CloudFormation
EC2 Free Tier Management with CloudFormation
Automating AWS Free Tier EC2 Management with CloudFormation
Managing cloud resources efficiently is essential, especially when working within the constraints of AWS's Free Tier. Unintended usage beyond the Free Tier limits can lead to unexpected charges, defeating the purpose of exploring AWS services at minimal cost. In this article, we'll explore how to automate the management of Amazon EC2 instances using AWS CloudFormation, ensuring you stay within Free Tier limits while optimizing your cloud usage.
Understanding the Basics
What Is Amazon EC2?

EC2
Amazon Elastic Compute Cloud (EC2) provides scalable computing capacity in the cloud, allowing you to run applications on virtual servers. These EC2 instances offer flexible compute power, making it easy to scale up or down based on your needs. You can choose from a variety of instance types tailored for different workloads, balancing CPU, memory, storage, and networking resources.
What Is AWS CloudFormation?
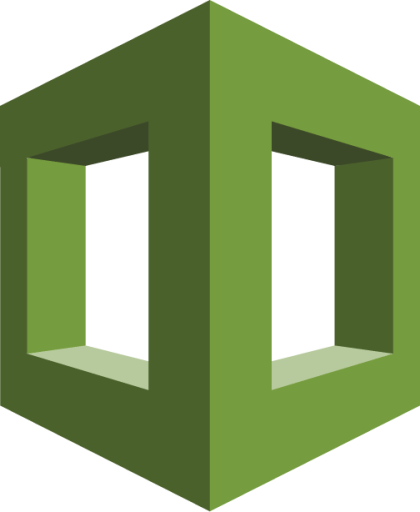
CloudFormation
AWS CloudFormation is a service that enables you to model and set up AWS resources using templates. These templates are text files written in JSON or YAML format that describe the desired state of your infrastructure. CloudFormation automates the provisioning and configuration of resources, ensuring consistent and repeatable deployments.
Why Managing the Free Tier Is Crucial

AWS offers a Free Tier that provides limited access to various services, including EC2, allowing new users to explore AWS without incurring costs. However, it's easy to unintentionally exceed these limits, leading to unexpected charges on your bill. By automating the management of Free Tier resources, you can prevent overuse, maintain cost efficiency, and focus on development rather than monitoring usage.
Automating EC2 Management with CloudFormation

Let's dive into how you can leverage AWS CloudFormation to automate the management of your EC2 instances, ensuring they stay within Free Tier limits.
Overview of the Solution
We'll create a CloudFormation template that sets up the following:
A Free Tier EC2 Instance: Configured with security measures to protect access.
A CloudWatch Alarm: Monitors the instance's CPU utilization.
A Lambda Function: Automatically stops the EC2 instance when CPU utilization exceeds a defined threshold.
IAM Roles and Policies: Grants necessary permissions while adhering to the principle of least privilege.
Key Features of the Template
Enhanced Security:
Security Groups: Restrict inbound traffic to specific ports and IP ranges.
IAM Policies: Provide only the necessary permissions for the Lambda function.
Resource Optimization:
Parameterized Inputs: Allow dynamic configuration based on user input.
Region Mappings: Automatically select the appropriate Amazon Machine Image (AMI) based on the deployment region.
Automated Monitoring:
CloudWatch Alarms: Continuously monitor EC2 instance metrics.
Lambda Function: Responds to alarms by stopping EC2 instances to prevent excessive usage.
The CloudFormation Template
Below is an excerpt of the CloudFormation template written in YAML. This template defines the resources and configurations needed to automate the management of your EC2 instance.
AWSTemplateFormatVersion: '2010-09-09'
Description: Automate EC2 Free Tier management with CloudFormation.
Parameters:
KeyPairName:
Type: AWS::EC2::KeyPair::KeyName
Description: Name of an existing EC2 KeyPair for SSH access.
Mappings:
RegionMap:
us-east-1:
AMI: ami-0c02fb55956c7d316
us-west-2:
AMI: ami-08962a4068733a2b6
Resources:
EC2Instance:
Type: AWS::EC2::Instance
Properties:
InstanceType: t2.micro
ImageId: !FindInMap [RegionMap, !Ref 'AWS::Region', AMI]
KeyName: !Ref KeyPairName
SecurityGroupIds:
- !Ref InstanceSecurityGroup
Tags:
- Key: Name
Value: FreeTierInstance
InstanceSecurityGroup:
Type: AWS::EC2::SecurityGroup
Properties:
GroupDescription: Enable SSH access
SecurityGroupIngress:
- IpProtocol: tcp
FromPort: 22
ToPort: 22
CidrIp: YOUR_IP_ADDRESS/32 # Replace with your IP address.
LambdaExecutionRole:
Type: AWS::IAM::Role
Properties:
AssumeRolePolicyDocument:
Version: '2012-10-17'
Statement:
- Effect: Allow
Principal:
Service:
- lambda.amazonaws.com
Action:
- sts:AssumeRole
Path: "/"
Policies:
- PolicyName: "LambdaEC2Policy"
PolicyDocument:
Version: '2012-10-17'
Statement:
- Effect: Allow
Action:
- ec2:DescribeInstances
- ec2:StopInstances
Resource: "*"
StopInstanceFunction:
Type: AWS::Lambda::Function
Properties:
Handler: index.lambda_handler
Role: !GetAtt LambdaExecutionRole.Arn
Code:
ZipFile: |
import boto3
def lambda_handler(event, context):
ec2 = boto3.client('ec2')
instance_id = event['detail']['instance-id']
ec2.stop_instances(InstanceIds=[instance_id])
Runtime: python3.9
Timeout: 30
CPUAlarm:
Type: AWS::CloudWatch::Alarm
Properties:
AlarmDescription: "Stop EC2 instance if CPU exceeds threshold"
Namespace: AWS/EC2
MetricName: CPUUtilization
Dimensions:
- Name: InstanceId
Value: !Ref EC2Instance
Statistic: Average
Period: 300
EvaluationPeriods: 1
Threshold: 70
ComparisonOperator: GreaterThanThreshold
AlarmActions:
- !GetAtt StopInstanceFunction.Arn
Outputs:
InstanceId:
Description: Instance ID of the EC2 instance
Value: !Ref EC2Instance
PublicDNS:
Description: Public DNS of the EC2 instance
Value: !GetAtt EC2Instance.PublicDnsName
Understanding the Template Components
Parameters: Allows users to input custom values when creating the stack, such as the key pair name.
Mappings: Associates AMIs with regions, enabling the template to select the correct image automatically.
Resources:
EC2Instance: The Free Tier eligible EC2 instance.
InstanceSecurityGroup: Controls inbound traffic to the EC2 instance.
LambdaExecutionRole: IAM role that grants permissions for the Lambda function.
StopInstanceFunction: The Lambda function that stops the EC2 instance.
CPUAlarm: CloudWatch alarm that monitors CPU utilization.
Outputs: Provides useful information after the stack is created, such as the instance ID and public DNS.
Deploying the CloudFormation Stack

Prerequisites
AWS Account: Ensure you have an active AWS account.
Permissions: You need sufficient permissions to create IAM roles, EC2 instances, Lambda functions, and CloudWatch alarms.
Steps to Deploy
Save the Template: Save the YAML content to a file named
ec2-management.yaml
.Access AWS CloudFormation Console: Log in to the AWS Management Console and navigate to the CloudFormation service.
Create a New Stack:
Click on Create stack and select With new resources (standard).
Choose Upload a template file and upload
ec2-management.yaml
.Click Next.
Specify Stack Details:
Stack Name: Provide a meaningful name for your stack.
Parameters: Enter your existing EC2 key pair name for the
KeyPairName
parameter.Click Next.
Configure Stack Options:
Configure tags, permissions, and advanced options as needed.
Click Next.
Review and Create:
Review all the details to ensure correctness.
Check the box acknowledging that AWS CloudFormation might create IAM resources.
Click Create stack.
Monitoring the Stack Creation
Stack Events: Monitor the progress in the Events tab.
Completion: Wait until the status changes to CREATE_COMPLETE.
Verifying and Testing the Setup

Accessing the EC2 Instance
Retrieve Outputs: In the CloudFormation stack's Outputs tab, find the
PublicDNS
.SSH Access:
ssh -i /path/to/your/key.pem ec2-user@PublicDNS
Test Connectivity: Ensure you can connect and the instance is running as expected. SSH into the EC2 instance
Conclusion
Automating the management of AWS Free Tier EC2 resources using CloudFormation empowers you to maintain control over your cloud environment, preventing unexpected costs and improving operational efficiency. By leveraging infrastructure as code, you ensure consistent deployments and can easily replicate environments as needed.